Since the first "big" prototype is to generate a month, the very first algorithm to create (or start in this case) is the actual Calendar-Table Generation. The 7x5 7x6 table.
To be as simple as possible for the first algorithm and prototype, we're going to predefine a month that starts on a Sunday. January 2006 started on a Sunday and is 31 days.
The algorithm is not going to have any calculation for dynamic generation. Today, I'm strictly putting down the basic algorithm for generating a 7x6 table, putting in the date, and stopping at 31.
Later, I will be using Structured English Pseudocode Standard. The reason is that it's easy to write, easy to decypher when coding, and easy to read and understand for anyone, even those that never programmed before. That's why I use this instead of other standards that gets too close to real programming. Not everyone can read code. (Also, I learned Structured French Pseudocode when I was in college, so there you go...)
But for now, I'm not going to go deep in the Pseudocode. We have to figure out the logic behind some elements here and there to even come up with a basic Pseudocode.
The first challenge I'm coming accross while working the algorithm is the weekly increment, without even thinking of the offsets yet. I know that I'm going to have two loops, one inside the other. The first to create the rows, and the other to create the columns inside the current row. The two loop indexes can be used to figure out a position.
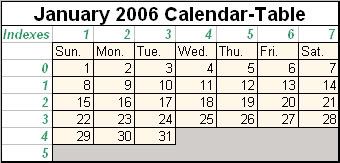
*Note: I'm using 1 for the starting column index for now. I think that's how I'll add the offset, but that's in the future. I will change it to 0+1 (see equation) if I need to in due time.
If you check a calendar for January 2006 and look at Monday the 9th, you are on the second column of the second row (row index 1). The preceding row, you had 2 on the same column because there are 7 days in a week. To figure out the day of any position on the table without any offsets, you just follow this simple equation (in Structured English Pseudocode):
currentDay ← columnIndex + (rowIndex * 7)
Validation:
On the calendar, the 5th falls on the first Thursday, the 18th falls on the third Wednesday, and the 21st on the third Saturday. With the grid above, let's fill in the equation:
5 ← 5 + (0 * 7)
18 ← 4 + (2 * 7)
21 ← 7 + (2 * 7)
It works! And there you have it. The first logic challenge I came across while working the algorithm solved and validated.